How to Build Responsive Mobile Apps with React Native Expertise
Are you ready to dive into the world of React Native and create stunning, responsive mobile apps? Look no further! In this comprehensive guide, we will walk you through the process of making your app truly responsive using React Native. Whether you're a seasoned developer or just starting out, this article will equip you with the knowledge and skills needed to craft exceptional user interfaces that adapt seamlessly across different devices. So, let's embark on this exciting journey of building a responsive app in React Native!
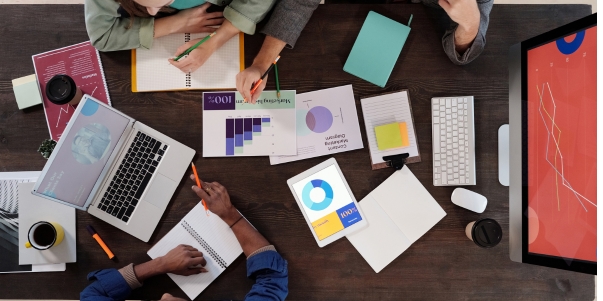
Responsive UI not only enhances user satisfaction but also improves engagement and retention
Understanding the Importance of Responsive UI
In today's mobile-first world, users expect applications to look and feel great on any device they use. A responsive user interface (UI) is crucial for providing an optimal experience regardless of screen size or orientation. With React Native, you can leverage its powerful features and components to create apps that automatically adjust and adapt to different devices, ensuring a consistent and visually appealing presentation.
Responsive UI not only enhances user satisfaction but also improves engagement and retention. By optimizing your app for responsiveness, you can reach a wider audience and maximize the impact of your application. So, let's explore how you can make your React Native app responsive and set it apart from the competition.
Setting Up Your Development Environment
Before we dive into the nitty-gritty of building a responsive app in React Native, let's ensure that you have a properly configured development environment. Here's a step-by-step guide to help you get started:
- Step One: Install Node.js and npm
- Step Two: Install the React Native CLI
- Step Three: Create a New React Native Project
- Step Four: Verify Your Setup
By following these steps, you'll have a solid foundation for developing your responsive app using React Native. Now, let's move on to mastering the art of building responsive UIs.
Building a Strong Foundation with Flexbox
Flexbox is a powerful layout system that plays a key role in creating responsive designs in React Native. It allows you to effortlessly distribute and align elements within containers, adapting to different screen sizes and orientations. With its intuitive approach, Flexbox makes it easier to create complex layouts while maintaining responsiveness.
To utilize Flexbox effectively, you need to understand its core concepts such as flex containers, flex items, and various positioning properties. By harnessing the power of Flexbox, you can achieve flexible and adaptive layouts that ensure your app looks great on any device.
Utilizing Media Queries for Device Adaptability
Media queries are essential tools for making your React Native app responsive across different devices. They allow you to apply specific styles based on the characteristics of the user's device, such as screen width, height, pixel density, and orientation. By leveraging media queries, you can fine-tune the appearance of your app and provide an optimal viewing experience for your users.
In React Native, you can use the Dimensions API to retrieve the device's screen dimensions dynamically. This information can then be utilized to conditionally apply styles or render different components based on the device's properties. With media queries, you can ensure your app adapts gracefully to various screen sizes and orientations.
Implementing Responsive Components
React Native offers a wide range of components that are designed to be responsive out of the box. By utilizing these components effectively, you can save time and effort in building responsive UIs. Some of the key responsive components in React Native include:
- Image: Use the
Image
component with appropriate props such asresizeMode
to ensure images adapt to different screen sizes while maintaining aspect ratio. - Text: Optimize text readability by adjusting font sizes based on the device's screen dimensions using media queries or dynamically calculated values.
- ScrollView: Allow users to scroll through content seamlessly on devices with limited screen space by wrapping your components within a
ScrollView
. - FlatList/SectionList: Render large lists efficiently while providing smooth scrolling by utilizing the performant
FlatList
orSectionList
components.
By leveraging these responsive components, you can build UIs that respond intelligently to various devices, delivering an exceptional user experience.
Enhancing User Experience with Dynamic Layouts
To take your app's responsiveness to the next level, consider incorporating dynamic layouts. A dynamic layout adjusts its structure based on the available screenreal estate. For example, you can display multiple columns in a wide screen layout and switch to a single column layout on smaller screens. This ensures that your app utilizes the available space efficiently and provides a seamless experience for users.
React Native offers various techniques for implementing dynamic layouts, such as:
- Conditional Rendering: Use conditional statements to render different components or layouts based on device properties or user interactions. For instance, you can conditionally render a sidebar navigation menu on larger screens and hide it on smaller screens.
- Platform-specific Components: React Native provides platform-specific components like
Platform.select
andPlatform.OS
that allow you to define different layouts or styles for specific platforms like iOS and Android. - Responsive Design Libraries: Consider leveraging responsive design libraries, such as
react-native-responsive-screen
, which provide utility functions to calculate dimensions and apply responsive styles based on the device's screen size.
By embracing dynamic layouts, you can create adaptable UIs that cater to the unique needs of different devices, enhancing the overall user experience.
Optimizing Performance for Responsiveness
While building a responsive app, it's crucial to ensure optimal performance to deliver a smooth and snappy user interface. Here are some key optimization techniques to consider:
- Virtualized Lists: When dealing with large lists, use virtualized list components like
FlatList
orSectionList
to efficiently render and recycle items as the user scrolls. This avoids performance issues caused by rendering an excessive number of elements at once. - Debouncing and Throttling: Implement debouncing and throttling techniques for resource-intensive tasks like fetching data from APIs or handling frequent user interactions. This helps reduce unnecessary renders and improves the overall responsiveness of your app.
- Code Splitting: Utilize code splitting techniques to load only the necessary components and resources when needed. This can significantly improve the initial loading time of your app, especially on slower networks or lower-end devices.
By employing these performance optimization strategies, you can ensure that your responsive app delivers a snappy and delightful user experience on a wide range of devices.
Testing and Debugging Your Responsive App
To guarantee the responsiveness and functionality of your React Native app across various devices, rigorous testing and debugging are essential. Here are some best practices to follow:
- Device Emulators/Simulators: Utilize device emulators or simulators to test your app on different screen sizes, resolutions, and operating systems. Platforms like Android Studio and Xcode provide powerful tools for simulating various devices.
- Real Devices: Test your app on real devices to verify its behavior in real-world conditions. This helps identify any device-specific quirks or performance issues that may not be apparent in emulated environments.
- Responsive Design Testing: Use tools like Chrome DevTools or the React Native Debugger to inspect and debug your app's responsive behavior. You can simulate different screen sizes, orientations, and network conditions to validate the responsiveness of your UI.
Thorough testing and debugging ensure that your app functions as intended on a wide range of devices, providing a seamless and responsive experience for your users.
Deploying Your App with Confidence
Once you have built and tested your responsive app in React Native, it's time to deploy it to the world! Here are some key steps to consider during the deployment process:
- App Store Guidelines: Familiarize yourself with the guidelines and requirements of the respective app stores (e.g., Apple App Store, Google Play Store) to ensure compliance and smooth submission.
- Optimized Builds: Generate optimized builds of your app by enabling minification, code obfuscation, and asset bundling. This helps reduce the app's size and improves performance.
- Release Channels: Consider utilizing release channels to control the rollout of updates to your app. Release channels allow you to gradually release new features or bug fixes to specific groups of users, reducing the risk of widespread issues.
- Monitoring and Analytics: Implement monitoring and analytics tools to track your app's performance, user engagement, and responsiveness. This data can help you identify areas for improvement and make informed decisions for future updates.
By following these deployment best practices, you can confidently launch your responsive React Native app and ensure a successful user experience.
Frequently Asked Questions (FAQs)
Q1: How can I make my React Native app responsive without compromising performance?
A: To make your app responsive while maintaining optimal performance, utilize techniques like virtualized lists, debouncing and throttling, and code splitting. These strategies help reduce unnecessary renders and optimize resource-intensive tasks.
Q2: Can I build different layouts for iOS and Android in React Native?
A: Yes, React Native provides platform-specific components that allow you to define different layouts or styles for specific platforms. You can use Platform.select
and Platform.OS
to conditionally render platform-specific UI elements.
Q3: Are there any libraries available to simplify responsive design in React Native?
A:A: Yes, there are several responsive design libraries available for React Native that can simplify the process. One popular library is react-native-responsive-screen
, which provides utility functions to calculate dimensions and apply responsive styles based on the device's screen size. By incorporating such libraries into your project, you can streamline the implementation of responsive UIs.
Q4: How can I test my responsive app in React Native on different devices?
A: You can test your responsive app on different devices using emulators or simulators offered by platforms like Android Studio and Xcode. These tools allow you to simulate various screen sizes, resolutions, and operating systems. Additionally, testing on real devices is recommended to validate your app's behavior under real-world conditions.
Q5: What are some performance optimization techniques for responsive apps in React Native?
A: Some key performance optimization techniques for responsive apps in React Native include utilizing virtualized lists, implementing debouncing and throttling for resource-intensive tasks, and employing code splitting to load only necessary components when needed. These optimizations help ensure a smooth and efficient user experience.
Conclusion
Congratulations! You have now mastered the art of creating a responsive app in React Native. By understanding the importance of responsive UI, setting up your development environment, harnessing the power of Flexbox, utilizing media queries, implementing responsive components, enhancing user experience with dynamic layouts, optimizing performance, and testing thoroughly, you can build exceptional mobile applications that adapt seamlessly across various devices.
Remember, responsiveness is a critical factor in providing an outstanding user experience and ensuring the success of your app. So embrace the techniques and best practices discussed in this article, and let your creativity and expertise shine through in crafting stunning and user-friendly mobile apps.
Now it's time to unleash your potential and create remarkable responsive apps that captivate users and make a lasting impact!