5 Levels of Object-Oriented Programming
Nearly all developers employ the core programming paradigm known as object-oriented programming (OOP) at some point in their careers. The most well-known programming paradigm, OOP, is taught as the norm for most of a programmer's educational career.
We'll dissect the fundamentals of what makes software object-oriented today so you can start using this paradigm for your tasks and job interviews.
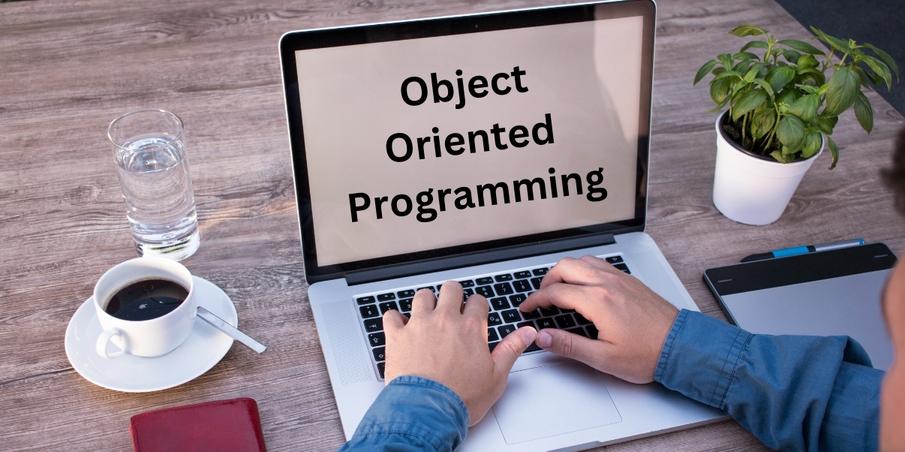
OOP is a programming style based on the idea of objects.
What is Object Oriented Programming(OOP)?
A programming paradigm known as object-oriented programming (OOP) is based on the ideas of classes and objects. It organizes software into straightforward, reusable classes of code blueprints, which are then utilized to build distinct instances of objects. The object-oriented programming languages JavaScript, C++, Java, and Python, are only a few examples.
Benefits of OOP
- OOP models complex things as reproducible, simple structures
- Reusable, OOP objects can be used across programs
- Allows for class-specific behavior through polymorphism
- Easier to debug, classes often contain all applicable information to them
- Secure protects information through encapsulation
Structure of OOP
The building blocks from OOP come in to help us do this by using Classes, Objects, Methods and Attributes.
Let's delve in and pinpoint the precise nature of these fundamental components:
- Classes serve as the building blocks for objects, properties, and methods. They are user-defined data types.
- Objects are instances of a class that have data that has been specifically defined. The description is the only item defined when a class is first created.
- Methods are described as internal class functions that explain an object's behavior. They are helpful for functionality that needs to be contained in one object at a time or for reusability. Reusable code is very helpful for debugging.
- Attributes: The state of an object is represented by its attributes, which are defined in the class template. Data is kept in the attribute field of objects.
Principles of OOP
Understanding the four OOP pillars we should follow will help us build good OOP code. These pillars are:
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
Let's dive in and better understand what exactly each of these means.
Encapsulation
This idea is what links the data together. Information is manipulated and kept secure by functions. If the information is concealed, no direct access is permitted to it. You must engage with the article that controls the information if you want to access it.
There's a reasonable probability that if you work for a firm, you've dealt with encapsulation. Consider a human resources division. The employees' data is contained (hidden) by the human resources team. They choose how this data will be handled and used. They must be consulted for all requests to access or update the worker data.
By encapsulating data, you increase the security and dependability of the information in your system. You can also keep an eye on who accesses the news and what actions are taken with it. This streamlines the debugging process and facilitates software maintenance.
Abstraction
Using straightforward classes to symbolize complexity is known as abstraction. Basically, we utilize abstraction to manage complexity by limiting the user's view to information that is pertinent and helpful. Driving an automated vehicle is a fantastic way to illustrate this.
If you have an automatic car, all you need to do to get from point A to point B is to start the vehicle after giving it the destination. After that, it will drive you there.
You don't need to understand how the car is built, how it correctly receives and executes commands, how it selects the best course of action from various possibilities, or any other technical details. When creating OOP apps, the same idea is used.
You accomplish this by obscuring information that the user doesn't need to view. Thanks to abstraction, your tasks can be managed more efficiently and in smaller, more manageable pieces.
Inheritance
Classes can take on characteristics from other classes through inheritance. As an illustration, you could group all cats together based on the fact that they share traits like having four legs. Their breeds further divide them into subcategories based on characteristics they share, like size and color.
In OOP, you utilize inheritance to group the objects in your applications based on their performance and shared traits. Because you can mix general features into a parent object and have child objects inherit these traits, interacting with the objects and programming is more accessible.
As an illustration, you might define an employee object that specifies all the general attributes of the employees in your business. Then, you'll be able to define a manager object with traits specific to managers in your organization while still inheriting features from the worker object. Any modifications to the worker object's implementation will be automatically reflected in the manager object.
Polymorphism
This is the ability of two unique items to respond to a single form. In order to avoid duplicating code, the program will identify which usage is essential for each execution of the thing from the parent class. Additionally, it enables various objects to communicate using the same interface.
Object-Oriented Programming Explained in 5 Levels of Difficulty
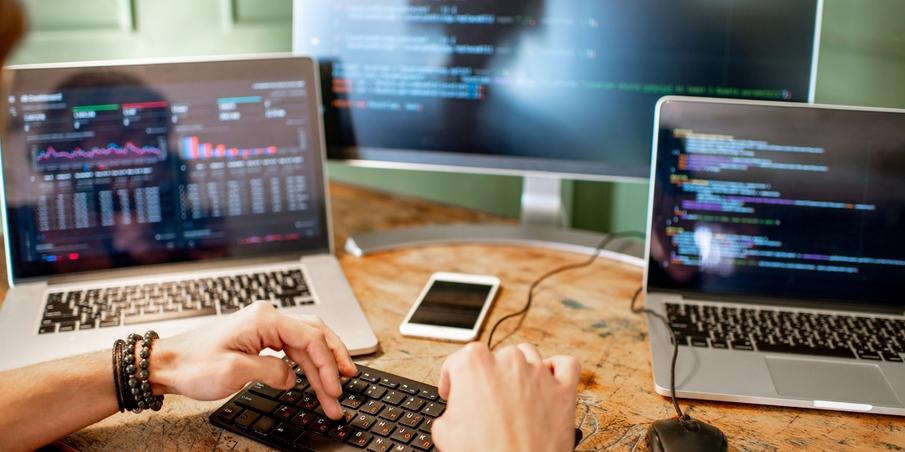
You can write code in many different ways
Child
Programming in the OOP fashion uses the concept of objects. A depiction of something in the real world is an object.
OOP makes it simple to develop software that can model actual objects. You may write programs that are simple to learn and comprehend using OOP.
Teen
There are numerous approaches to writing code. OOP, or object-oriented programming, is one of the most well-known and widely used. The OOP programming approach is derived from the idea of objects. These things can communicate with one another. For the objects to cooperate, we write the code in a particular way.
OOP provides several benefits over other programming paradigms. One advantage is that since the code is more declarative (what we require) rather than imperative, it is simpler to read and comprehend (how we do it).
By looking at the OOP code, you can tell which objects are being utilized and how they relate. As opposed to when you were looking at a large number of unrelated lines of code, this makes it much simpler to understand what the code is doing.
The fact that OOP increases code reuse is another benefit. If you have created a valuable piece of code, you can reuse it in another program without having to duplicate it.
College Student
The programming methodology known as object-oriented programming (OOP) is built on the idea of objects and their behavior.
There are two methods by which we can specify how an object behaves:
- Prototyping: By imitating other things, we can construct new ones.
- Classifying: To build new objects, we use templates known as classes that specify the properties of the new stuff.
Compared to procedural programming, OOP code is frequently written more understandably and naturally. Additionally, it enables programmers to build programs using reusable parts.
For instance, we don't need to rewrite an object representing a bank account multiple times in different programs.Its potential for being more challenging to learn than other programming paradigms is a drawback.
The syntax (the rules for writing the code) can be complicated for beginners. Another possible disadvantage is that OOP programs often execute more slowly than those written in low-level languages.
Previously, keeping track of all the objects and their interactions required additional overhead. Today, unless you're working on large or urgent projects, this difference doesn't matter.
OOP might be the appropriate choice for you if you're interested in building software that is simple to read and reuse or if you want to be able to make modifications to your applications without starting from scratch.
Grad Student
The goal of object-oriented programming (OOP), a type of programming, is to create programs by utilizing objects. Each object is a distinct entity generated, modified, and destroyed independently in object-oriented programming.
The foundation of OOP is the idea that objects should behave like their actual counterparts. Objects can be derived from other objects or made entirely from scratch. Once we develop an item, there are countless applications for it. A class system is generally used in OOP languages to define objects.
A class describes an object's collaborators and behavior, acting as a kind of blueprint for the object. Since every object is an instance of a class, they all share the same structure and behavior.
Expert
The programming method known as “object-oriented programming,” or simply “OOP,” is founded on the idea of objects. Typically, these items are grouped into classes that can be used to create unique instances of the class (called objects). Software that is easy to understand and maintain can be created using OOP.
OOP may reduce the amount of duplicate code, which is one of its advantages. It is simple to duplicate code when writing new functions or modules when utilizing a procedural programming method. This can cause maintenance problems in the future if we need to modify one piece of duplicated code because we would also need to modify all other instances of the code. OOP can provide more robust, more dependable code.
Conclusion
Today, most programming languages allow programmers to combine different paradigms. This frequently occurs because different types of programming techniques will employ them.
Most OOP developers concur that employing it generally results in superior data structures and code reusability. Long-term time savings result from this.
We at Groove Technology are willing to help you develop your idea with object-oriented programming, be sure to contact us here to learn how we can assist.