How to Create a React Native Library: A Beginner’s Guide
6 min readIf you're looking to create your own library for use in a React Native project, you've come to the right place. In this tutorial, we'll walk you through the steps of how to create a React Native library from scratch, including all the tools and resources you'll need along the way.
What is a React Native Library?
A React Native library is a collection of reusable components that can be incorporated into any React Native project, just like any other library. This approach allows developers to save time by reusing code rather than having to write it from scratch every time.
Getting Started: Tools and Resources
Before you begin creating your React Native library, you'll need to set up your development environment. Here are some of the tools and resources you'll need:
- Node.js
- Yarn or NPM
- React Native CLI
- An IDE (such as Visual Studio Code)
Once you have these tools installed on your machine, you're ready to start creating your library.
Step-by-Step Guide: Creating a React Native Library
Step 1: Initialize Your Project
The first step in creating your React Native library is to initialize your project with the create-react-native-library
tool. This utility will generate a basic structure for your library, including a sample component and some boilerplate files.
To initialize your project, run the following command in your terminal window:
npx create-react-native-library my-library
This will create a new folder called my-library
in your working directory.
Step 2: Customize Your Library
Once you've initialized your project, you can start customizing your library by adding your own components. To create a new component, simply add a new file to the src
folder with the .js
extension.
For example, let's say you wanted to create a button component. You would create a new file in the src
folder called Button.js
. This file would contain the code for your button component.
Step 3: Build Your Library
Once you've added all of your components to your library, it's time to build it. To build your library, run the following command:
npm run build
This will generate a lib
folder in your project directory with the compiled version of your library.
Step 4: Publish Your Library
The final step in creating your React Native library is to publish it to NPM so that others can use it in their projects. To do this, you'll need to create an account on the NPM website and then run the following command:
npm publish
This will publish your library to the NPM registry, making it available for anyone to install and use in their own projects.
Examples of React Native Libraries
There are countless examples of React Native libraries available on NPM that you can use as inspiration for your own projects. Some popular libraries include:
- React Navigation
- React Native Elements
- React Native Paper
- React Native Maps
- React Native Firebase
All of these libraries provide useful components that can be easily incorporated into any React Native project.
Comparing React Native Libraries
When choosing a React Native library for your project, there are a few factors to consider. First, you'll want to make sure that the library provides the components you need for your project. You'll also want to look for a library with good documentation, active development, and a large user base.
Some popular React Native libraries, such as React Navigation and React Native Elements, have all of these qualities, making them excellent choices for many projects. However, the best choice for your project may depend on your specific needs and preferences.
Advice for Creating a React Native Library
When creating your own React Native library, it's important to keep a few things in mind. First, you'll want to create components that are as reusable and flexible as possible, so that they can be easily incorporated into any project.
You should also focus on providing clear documentation and examples, so that others can easily understand how to use your library. Additionally, it's important to stay up to date with the latest trends and best practices in React Native development, so that your library remains relevant and useful over time.
FAQs
Q: Can I create a React Native library without using the create-react-native-library
tool?
A: Yes, it's possible to create a React Native library from scratch without using the create-react-native-library
tool. However, this can be more challenging and time-consuming than using the tool.
Q: How do I test my React Native library?
A: You can test your React Native library using tools like Jest or Enzyme, which allow you to write unit tests for your components.
Q: Can I use TypeScript to create a React Native library?
A: Yes, you can use TypeScript to create a React Native library. In fact, TypeScript is becoming an increasingly popular choice for many React Native developers, thanks to its strong type checking and other features that help make development faster and more error-free.
To create a React Native library with TypeScript, you can follow the same steps outlined in this tutorial, but add the --template typescript
flag when initializing your project with create-react-native-library
. This will generate a TypeScript-based project structure for your library.
Q: Can I use my React Native library in other frameworks besides React Native?
A: While React Native libraries are designed specifically for use in React Native projects, it's possible to use them in other frameworks as well. However, this may require additional configuration and setup, depending on the framework in question.
Q: What should I do if I encounter bugs or issues with my React Native library?
A: If you encounter bugs or issues with your React Native library, you should first check the documentation and GitHub repository for the library to see if there are any known solutions or workarounds. If not, you can open an issue on the repository to report the problem and seek assistance from the library's maintainers.
Q: How can I promote my React Native library to others?
A: Once you've created your React Native library, you can promote it to others by publishing it on NPM, sharing it on social media and developer forums, and including it in any relevant blog posts or tutorials you write. You can also contribute to open-source React Native projects and engage with the community to help build awareness of your library.
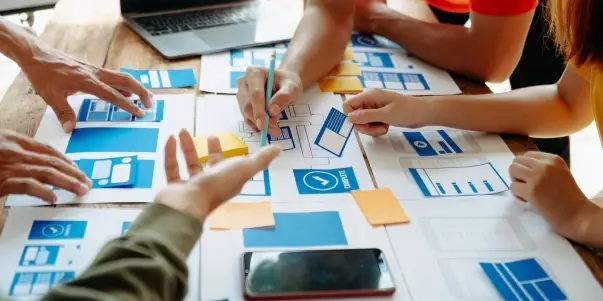
Creating a React Native library can be a rewarding experience
Conclusion
Creating a React Native library can be a rewarding experience that allows you to share your knowledge and skills with others. By following the steps outlined in this tutorial and staying up to date with the latest trends and best practices in React Native development, you can create a high-quality library that others will find useful and valuable.
Remember to focus on creating reusable and flexible components, providing clear documentation and examples, and staying engaged with the React Native community. With these tips in mind, you'll be well on your way to creating a successful React Native library of your own. If not, please feel free to get in touch with our experienced developers at Groove Technology!