How to Create a Single Page Application Using ReactJS
Building a single-page application (SPA) using ReactJS can initially seem daunting, especially for beginners. However, with the right approach and understanding, you can create a powerful and efficient web application that provides a seamless user experience. This article will explore the step-by-step process of creating a single-page application using ReactJS, along with examples, comparisons, and expert advice.
Understanding Single Page Applications
Before diving into the technical aspects of building a single-page application using ReactJS, let's define what exactly a single page application is. A single page application is a web application that dynamically updates the content on the page without requiring a full page reload. Instead, it fetches data from the server and updates the view on the client side.
Compared to traditional multi-page applications, single page applications offer a smoother user experience by eliminating the need for page refreshes. They provide better performance, improved interactivity, and allow for the creation of more complex and interactive interfaces.
Setting Up the Development Environment
To start building a single page application using ReactJS, you need to set up your development environment. Here are the steps you should follow:
- Install Node.js: Node.js is required to run JavaScript code outside of a web browser. Download and install the appropriate version of Node.js for your operating system.
- Create a New React Project: Open your terminal or command prompt and navigate to the desired directory. Run
npx create-react-app my-app
to create a new React project named “my-app”. - Navigate to the Project Directory: Use the
cd my-app
command to enter the project directory. - Start the Development Server: Execute
npm start
to start the development server. This will open your application in a web browser athttp://localhost:3000
.
Building the User Interface with React Components
One of the key aspects of building a single page application using ReactJS is breaking down your user interface into reusable components. React components are independent and self-contained units of UI that can be combined to create complex interfaces. Here's how you can create and use React components:
- Create a New Component: In the “src” directory of your project, create a new file with a
.js
extension. Inside the file, define your component using theclass
orfunction
syntax. - Import and Use Components: To use a component in another component, import it using the
import
statement at the top of your file. You can then use the imported component as if it were an HTML tag.
import React from 'react';
import MyComponent from './MyComponent';
function App() {
return (
Hello, World!
);
}
export default App;
Routing in Single Page Applications
Routing is crucial for navigating between different views in a single page application. React Router is a popular library that provides routing capabilities in ReactJS applications. Follow these steps to set up routing in your single page application:
- Install React Router: Execute the command
npm install react-router-dom
to install React Router in your project. - Define Routes: Import the necessary components from React Router and define routes inside your main component. Map each route to a specific React component.
- Implement Navigation: Use the component provided by React Router to create navigation links. Place these links wherever navigation is required in your application.
import from 'react-router-dom';
function App() {
return (
Home
About
);
}
Managing State with React Hooks
State management is an essential part of building a single page application, especially when dealing with user interactions and data fetching. React Hooks, introduced in React 16.8, provide a way to manage state in functional components. Here's an example of how to use React Hooks for state management:
“`jsx import React, from ‘react';
function Counter() { const [count, setCount] = useState(0);
return (
Count:
setCount(count
Examples of Creating a Single Page Application Using ReactJS
To better understand the process of creating a single page application using ReactJS, let's explore some examples:
- To-Do List Application: A common example is a to-do list application where users can add, edit, and delete tasks. React components like
TaskList
,TaskItem
, andAddTaskForm
can be created to manage the UI and state of the application.
- Weather Forecast Application: Another example is a weather forecast application that displays weather information for different locations. React components such as
LocationSelector
,WeatherCard
, andWeatherDetails
can be used to fetch and display weather data dynamically.
- E-commerce Product Catalog: Building an e-commerce product catalog with filtering and sorting options is another interesting use case. React components like
ProductList
,FilterBar
, andSortDropdown
can be implemented to provide a seamless shopping experience.
Comparisons of Single Page Applications with Traditional Web Applications
When considering whether to build a single page application or a traditional web application, it's essential to evaluate their advantages and disadvantages:
- Performance: Single page applications tend to have faster initial load times since only the required data is fetched from the server. Traditional web applications often require full page reloads, leading to slower loading times.
- User Experience: Single page applications provide a smoother user experience with instant transitions between pages. Traditional web applications may feel clunky and less responsive due to frequent page refreshes.
- Development Complexity: Single page applications can be more complex to develop compared to traditional web applications. They require knowledge of frameworks like ReactJS and additional libraries for routing, state management, etc.
- Search Engine Optimization (SEO): Traditional web applications are generally easier to optimize for search engines since each page has its own unique URL. Single page applications require extra effort to ensure proper SEO implementation.
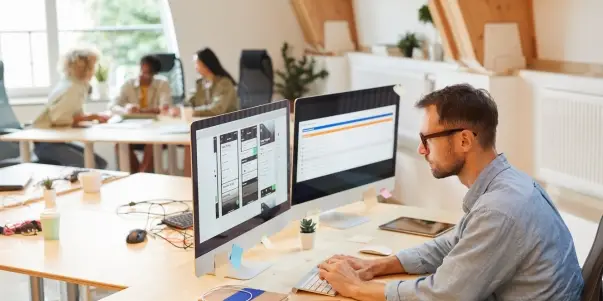
ReactJS can be used for projects of any size
Expert Advice for Building Single Page Applications Using ReactJS
As you embark on the journey of building a single page application using ReactJS, here are some expert tips to keep in mind:
- Component Reusability: Aim for maximum component reusability to reduce code duplication and improve maintainability. Break down your UI into smaller, reusable components that can be combined to create complex interfaces.
- State Management: Choose an appropriate state management solution based on your application's complexity. React Hooks, like
useState
anduseEffect
, provide simple state management for small to medium-sized applications. For larger applications, consider using libraries like Redux or MobX.
- Performance Optimization: Pay attention to performance optimization techniques such as code splitting, lazy loading, and caching. These techniques can significantly improve the speed and responsiveness of your single page application.
- Testing: Write comprehensive unit tests for your React components using testing frameworks like Jest and Enzyme. Automated testing ensures that your application functions as expected and makes future refactoring and feature additions easier.
- Continuous Learning: Keep up with the latest ReactJS updates, best practices, and community trends. The JavaScript ecosystem is continually evolving, and staying updated will help you build better single page applications.
Frequently Asked Questions (FAQs)
Q1: Can I use ReactJS for small projects or personal websites?
A1: Absolutely! ReactJS can be used for projects of any size. It provides benefits like component reusability and a fast development workflow, making it a great choice even for small projects or personal websites.
Q2: Are single page applications suitable for all types of websites?
A2: While single page applications offer several advantages, they may not be suitable for every type of website. Websites heavily dependent on search engine optimization or those with a significant amount of static content may benefit more from traditional web applications.
Q3: Can I use other JavaScript frameworks with ReactJS? A3: Yes, you can combine ReactJS with other JavaScript frameworks or libraries. React's flexible architecture allows it to work seamlessly alongside frameworks like Angular or Vue.js in a hybrid setup.
Q4: How can I handle form submissions in a single page application?
A4: In a single page application, form submissions can be handled by making API calls to the server using libraries like Axios or the built-in fetch
API. Upon successful submission, you can update the UI accordingly.
Q5: Is it necessary to learn JavaScript before diving into ReactJS?
A5: It is highly recommended to have a solid understanding of JavaScript fundamentals before starting with ReactJS. Knowledge of JavaScript concepts like variables, functions, and arrays will greatly facilitate your learning process.
Conclusion
Building a single page application using ReactJS offers numerous benefits, such as improved performance, seamless user experience, and better code maintainability. You can create powerful and dynamic web applications by following the steps outlined in this article. Or you can also get the professional support from the developers at Groove Technology!