React Hooks: Simplifying State Management in React Native
React Native has revolutionized mobile app development by allowing developers to use the same codebase for both iOS and Android apps. However, managing state in React Native can be challenging, especially when dealing with complex components. This is where React Hooks come in – a game-changer that simplifies state management in React Native.
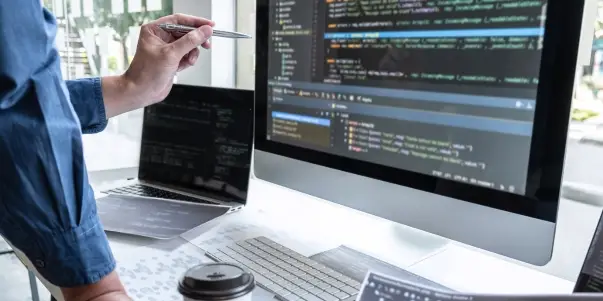
React Native has revolutionized mobile app development
What are Hooks in React Native?
Hooks are functions that allow you to use React features such as state and life-cycle methods inside functional components. Before hooks, it was necessary to use class components to manage component state. Class components are more verbose and harder to read than functional components. Hooks have made it easier to write and read React code.
What are React Hooks and Why do we Need Them?
React Hooks are a set of functions introduced in React 16.8 that allow you to use state and other React features in functional components. Hooks eliminate the need for class components, which can make your code more concise and more comfortable to read.
Functional components are lightweight and easy to understand because they don't have the complex life-cycle methods of class components. With Hooks, developers can now use functional components to manage state and react to user interactions.
Why Are React Hooks Called “Hooks”?
The name “Hooks” comes from the way these functions allow you to “hook” into React's core functionality. By calling a Hook, you can add state to a functional component, or use other React features like context or effects.
Why Do We Use Hooks in React?
Hooks are a fundamental feature of React that were introduced in version 16.8. They enable developers to use state, lifecycle methods and other React features within functional components, which previously could only be done with class components. In this way, they offer a more concise and flexible way to build complex UIs.
One of the primary reasons why we use hooks in React is to manage state. In React, state is an object that stores data that can change over time and triggers re-rendering when it updates. Prior to the introduction of hooks, state could only be managed in class components via the constructor and the setState method.
However, functional components didn't have access to these methods, which forced developers to choose between either using class components or employing workarounds like Higher-Order Components (HOCs) or Render Props patterns. Hooks provide a simpler way to use state in functional components by allowing us to declare and update it directly from within the component function body, using the useState hook.
Hooks also allow us to handle side effects and lifecycle methods in functional components. Side effects are any code that interacts with the outside world, such as fetching data, updating the DOM or setting timeouts. Previously, using lifecycle methods like componentDidMount and componentDidUpdate was the only way to handle side effects in React. However, this approach has its limitations since lifecycle methods are only available in class components.
With the useEffect hook, we can define side effects in functional components too. The useEffect hook runs after every render and allows us to perform actions such as fetching data from APIs, subscribing and unsubscribing to event listeners, setting up and clearing timeouts and intervals, etc.
Another advantage of hooks is that they enable the creation of custom reusable logic. Custom hooks are functions that encapsulate specific behavior and can be used across multiple components. This means we can create our own hooks to encapsulate commonly used functionality such as form validation, API requests, or authentication logic, and then reuse it across multiple components in our application.
In summary, we use React hooks to simplify the process of managing state, handling side effects and lifecycle methods, and creating reusable logic in functional components. These features make it easier for developers to write cleaner, more concise, and maintainable code, while also making it possible to build complex UIs with functional components.
How Do React Hooks Work?
React Hooks work by providing a set of built-in functions that allow you to use state, context, and other React features inside functional components. Here are some of the most commonly used Hooks:
useState
The useState
Hook allows functional components to manage state. It returns an array with two values – the current state value and a function to update that value.
import React, from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
You clicked times
setCount(count + 1)}>
Click me
);
}
In this example, we use the useState
Hook to define a state variable called count
. We also create a function called setCount
which can be used to update the count
value.
useEffect
The useEffect
Hook allows you to perform side effects inside functional components. It replaces the lifecycle methods in class components.
import React, from 'react';
function Counter() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked $ times`;
});
return (
You clicked times
setCount(count + 1)}>
Click me
);
}
In this example, we use the useEffect
Hook to update the document title whenever the count
value changes.
useContext
The useContext
Hook allows you to access data from React's context API inside functional components.
import React, from 'react';
import from './ThemeContext';
function Title() {
const theme = useContext(ThemeContext);
return (
Hello, World!
);
}
In this example, we use the useContext
Hook to get the current theme value from the ThemeContext
.
FAQs
Q. How do I create custom Hooks?
- You can create custom hooks by using existing React hooks and encapsulating the logic in a function. Here is an example of a custom hook:
import from 'react';
export function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
async function fetchData() {try {
const response = await fetch(url);
const json = await response.json();
setData(json);
setLoading(false);
} catch (error) {
console.error(error);
setLoading(false);
}
}
fetchData();
return ;
}
This custom hook uses the useState
and useEffect
Hooks to fetch data from an API.
Q. Can I use Hooks in class components?
- No, Hooks can only be used in functional components.
Q. Are Hooks compatible with React Native?
- Yes, Hooks are fully compatible with React Native. In fact, Hooks were introduced in React 16.8, which is included in React Native 0.59 and later.
Q. What is the difference between useState
and useReducer
?
- Both
useState
anduseReducer
allow you to manage component state, butuseReducer
is more suitable for complex state logic.useReducer
works by dispatching actions to update state, whereasuseState
simply updates state directly.
Q. Are there any performance drawbacks to using Hooks?
- No, Hooks do not have any performance drawbacks compared to class components. In fact, Hooks can improve performance in certain cases by reducing unnecessary re-renders.
Conclusion
React Hooks have made state management in React Native much simpler and more intuitive. By allowing us to use state and other React features inside functional components, Hooks have eliminated the need for class components and made code more readable and concise. Whether you're a seasoned React developer or just getting started, learning how to use Hooks is a crucial skill that will make your code more efficient and effective.
Contact Groove Technology today for further consultation!