Journey to Becoming a Successful React Native Mobile Developer
Are you passionate about mobile app development and looking to dive into the world of React Native? If so, you've come to the right place! In this comprehensive guide, we will explore the exciting realm of React Native and provide you with all the insights you need to embark on a successful journey as a React Native mobile developer. Whether you're an experienced native developer or completely new to mobile app development, this article will equip you with the knowledge, skills, and resources necessary to thrive in the ever-expanding world of React Native.
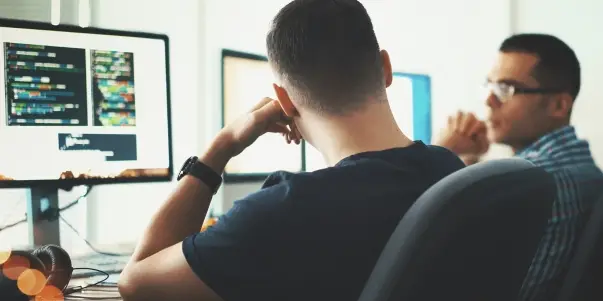
The role of a React Native mobile developer involves various responsibilities
Understanding the Role of a React Native Mobile Developer
A React Native mobile developer is a software engineer who specializes in building mobile applications using the React Native framework. React Native is a popular open-source framework developed by Facebook that allows developers to build cross-platform mobile apps using JavaScript and React.
The role of a React Native mobile developer involves various responsibilities throughout the app development lifecycle. Here is a detailed explanation of their role:
- Application Development: A React Native developer is responsible for developing mobile applications using the React Native framework. They work closely with the UI/UX designers and backend developers to understand the requirements and implement the user interface and features of the app. They write code in JavaScript and use React Native components to create interactive and responsive user interfaces.
- Cross-Platform Development: One of the key advantages of using React Native is its ability to build apps that work on both iOS and Android platforms. A React Native developer leverages this capability to write code once and deploy it on multiple platforms. They ensure that the app's functionality and user experience remain consistent across different devices and operating systems.
- Code Optimization and Performance: React Native developers are responsible for optimizing the codebase to enhance the performance of the mobile app. They identify and resolve performance bottlenecks, memory leaks, and other issues that may affect the app's speed and responsiveness. They also stay up-to-date with the latest best practices and tools to improve the overall performance of the application.
- Integration with Backend Services: Mobile applications often require integration with various backend services such as APIs, databases, authentication systems, and cloud storage. A React Native developer works with backend developers to establish these integrations, ensuring seamless communication between the app and the server. They handle data fetching and synchronization, implement authentication mechanisms, and handle error handling and data validation.
- Testing and Debugging: React Native developers are responsible for testing and debugging the mobile app to ensure its functionality and reliability. They perform unit testing, integration testing, and end-to-end testing to identify and fix any issues or bugs in the application. They use debugging tools and techniques to trace and resolve errors, ensuring a smooth user experience.
- Collaboration and Communication: React Native developers work closely with other team members, including designers, product managers, and backend developers. They actively participate in meetings and discussions to understand project requirements, provide technical insights, and collaborate on app development tasks. Effective communication skills are crucial for conveying ideas, discussing challenges, and finding solutions as part of a development team.
- Continuous Learning and Improvement: The field of mobile app development is constantly evolving, and a React Native developer must stay updated with the latest trends, frameworks, and technologies. They engage in continuous learning, attend relevant conferences or webinars, and explore new tools and libraries to enhance their skills and improve the quality of their work. They also actively contribute to the React Native community by sharing knowledge and resources.
Getting Started as a React Native Mobile Developer
Now that we've covered the basics, let's dive into the practical steps to kickstart your journey as a React Native mobile developer:
Familiarize Yourself with JavaScript and React Concepts
To become proficient in React Native, having a strong foundation in JavaScript is crucial. Make sure to grasp the fundamentals of JavaScript programming, including variables, functions, objects, and control flow. Additionally, understanding React's core concepts such as components, state, and props will provide a solid basis for working with React Native.
Set Up Your Development Environment
To develop React Native applications, you'll need to set up your development environment. Install Node.js, which includes the Node Package Manager (npm) used for managing dependencies. Additionally, install a code editor of your choice, such as Visual Studio Code or Atom, to write and edit your React Native code.
Learn the React Native Fundamentals
Start by understanding the basic building blocks of React Native, including components, JSX (JavaScript XML), and styling techniques. Dive into the documentation and explore the core components provided by React Native, such as View, Text, Image, and TouchableOpacity.
Navigation is a crucial aspect of mobile app development. Familiarize yourself with popular navigation solutions for React Native, such as React Navigation or React Native Navigation. These libraries provide easy-to-use and customizable navigation options for your applications.
Build Projects and Learn by Doing
While theoretical knowledge is essential, hands-on experience is key to mastering React Native. Start building small projects, experiment with different features and libraries, and challenge yourself with progressively more complex applications. Learning by doing will help solidify your understanding and prepare you for real-world scenarios.
Building your first React Native application
Building your first React Native application can be an exciting and rewarding experience. React Native is a popular framework that allows you to build cross-platform mobile applications using JavaScript and React. It combines the best aspects of native development with the simplicity and flexibility of web development.
To get started, you'll need to set up your development environment. Make sure you have Node.js installed on your machine, as well as a code editor like Visual Studio Code or Atom. You'll also need to install the React Native CLI globally by running the command npm install -g react-native-cli
.
Once your environment is set up, you can create a new React Native project by running react-native init MyApp
. This will create a new directory called “MyApp” with a basic project structure.
Next, navigate into the project directory by running cd MyApp
and start the development server with the command npm start
or yarn start
. This will launch the Metro Bundler, which bundles and serves your JavaScript code to your app.
Now, it's time to run your app on a simulator or a physical device. If you're using macOS, you can run the iOS simulator by executing npx react-native run-ios
. For Android, open an emulator or connect a physical device and run npx react-native run-android
. This will compile your app and deploy it to the selected platform.
At this point, you should see the default React Native app running on your simulator or device. Congratulations! You've successfully built and launched your first React Native application.
To start customizing your app, open the project in your preferred code editor. The entry point for your app is typically index.js
, where you can import and register your custom components. React Native uses a component-based architecture, so you'll be building reusable UI components that make up your app.
You can create a new component by creating a new JavaScript file, such as MyComponent.js
, and defining your component using the React Native APIs. These APIs allow you to create UI elements like text, buttons, images, and more. You can style your components using either inline styles or external style sheets.
To navigate between screens in your app, you'll need to use a navigation library like React Navigation. This library provides a set of navigators that handle routing and screen transitions. Install it by running npm install react-navigation
or yarn add react-navigation
.
Once installed, you can define your app's navigation structure by creating a new file, such as AppNavigator.js
, where you'll set up different screens and their corresponding routes. You can then import and use this navigator in your index.js
file to enable navigation in your app.
As you continue building your app, you'll likely need to interact with data from an API or a local database. React Native provides several options for handling data, including libraries like Axios for making HTTP requests and AsyncStorage for storing data locally.
Throughout the development process, you can test your app on both iOS and Android platforms, allowing you to ensure consistent behavior across different devices. React Native provides access to platform-specific APIs, so you can leverage device features like geolocation, camera, and push notifications.
When you're ready to distribute your app, you can generate release builds for both iOS and Android. For iOS, you'll need to create an Apple Developer account and follow the necessary steps to generate an IPA file or upload your app to the App Store. Similarly, for Android, you'll need a Google Developer account and follow the procedures to generate an APK file or publish your app on the Google Play Store.
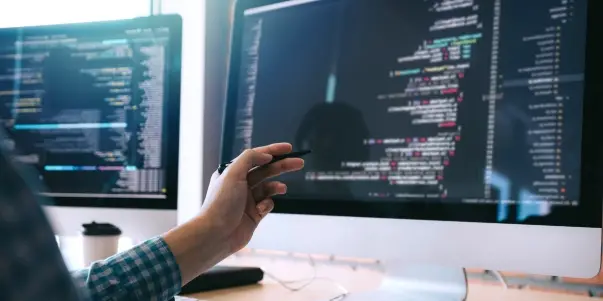
Throughout the development process, you can test your app on both iOS and Android platforms
Mastering Essential React Native Concepts
Mastering Essential React Native Concepts involves gaining a comprehensive understanding of the fundamental principles, components, and techniques used in developing mobile applications using the React Native framework. React Native is a popular JavaScript library that enables developers to build native mobile apps for both iOS and Android platforms using a single codebase.
To begin mastering React Native concepts, it is essential to have a solid foundation in React, as React Native builds upon the same principles and syntax. React Native utilizes a declarative programming approach, allowing developers to describe the desired user interface and let the framework handle the rendering logic.
One crucial concept in React Native is components. Components are the building blocks of any React Native application. They encapsulate reusable pieces of user interface and behavior, providing a modular and structured approach to development. React Native offers a rich set of pre-defined components such as View, Text, Image, Button, and TextInput, which can be combined and customized to create complex UI elements.
Styling is another vital aspect of React Native development. React Native employs a flexbox-based layout system similar to CSS, enabling developers to define the positioning, sizing, and alignment of UI components. Additionally, React Native supports StyleSheet, a style abstraction that helps separate the styling concerns from the component's logic.
React Native also provides a powerful mechanism for handling user interactions through touch events and gestures. Developers can capture user input using touchable components like TouchableOpacity, TouchableHighlight, or TouchableWithoutFeedback. Furthermore, React Native integrates gesture recognition APIs, allowing developers to implement swipes, pinches, and other complex gestures in their applications.
Asynchronous data handling is a common requirement in mobile development, and React Native provides several mechanisms to address this. The framework supports network requests using the Fetch API or third-party libraries like Axios. It also facilitates local data management by offering the AsyncStorage API for persistent storage and state management solutions like Redux or MobX.
Navigation is an essential concept in any mobile application. React Native offers various navigation libraries such as React Navigation and React Native Navigation, which allow developers to implement intuitive and efficient navigation flows within their apps. These libraries provide a range of navigators, including stack navigator, tab navigator, drawer navigator, and more.
React Native enables the integration of native device features through the use of Native Modules and Native UI Components. This allows developers to access device functionalities like camera, geolocation, contacts, sensors, and more. By bridging the gap between JavaScript and platform-specific code, React Native provides a way to leverage the full capabilities of the underlying operating systems.
Debugging and testing are crucial for ensuring the quality and stability of React Native applications. React Native integrates with popular debugging tools such as Chrome DevTools or React Native Debugger, enabling developers to inspect and debug their JavaScript code. Additionally, React Native provides tools like Jest for unit testing and Detox for end-to-end testing.
Deployment is the final step in the React Native development process. To distribute apps to the App Store or Play Store, developers need to configure the necessary project settings, generate the appropriate certificates and provisioning profiles, and utilize build tools like Xcode or Android Studio. React Native simplifies this process by providing detailed documentation and guidelines for each platform.
Frequently Asked Questions (FAQs)
**FAQ 1: What skills do I needto become a successful React Native mobile developer?
Answer: As a React Native mobile developer, having a combination of technical skills and problem-solving abilities is crucial. Here are the key skills you need to focus on:
- Proficiency in JavaScript: Since React Native is built on JavaScript, a strong understanding of the language is essential. Familiarize yourself with concepts like ES6 syntax, asynchronous programming, and functional programming.
- Knowledge of React and React Native: Understanding React's core principles, including components, state management, and lifecycle methods, is fundamental. Learn how to apply these concepts specifically to React Native development.
- Familiarity with native mobile development: While React Native abstracts away many platform-specific details, having some knowledge of iOS and Android development will be beneficial. This includes understanding native UI components, platform-specific APIs, and app deployment processes.
- Experience with version control: Git is a widely used version control system in the software development industry. Being proficient in using Git for code collaboration and managing project versions will greatly enhance your productivity as a React Native developer.
- Problem-solving and debugging skills: Mobile app development often involves encountering challenges and bugs. Being able to analyze problems, troubleshoot effectively, and find solutions is a valuable skill set. Familiarize yourself with debugging tools and techniques specific to React Native.
FAQ 2: Can I use existing web development skills for React Native?
Answer: Absolutely! If you have experience in web development, particularly with React, you can leverage your existing skills when learning React Native. React Native utilizes similar concepts and patterns as React, such as component-based architecture and JSX syntax. While there may be some platform-specific nuances to learn, your familiarity with React will give you a head start in understanding React Native's core principles.
FAQ 3: Are there any resources or tutorials to help me learn React Native?
Answer: Yes, there are plenty of resources available to help you learn React Native. Here are some recommendations:
- Official Documentation: Start by exploring the official React Native documentation, which provides comprehensive guides, API references, and tutorials.
- Online Courses and Tutorials: Platforms like Udemy, Coursera, and Pluralsight offer a wide range of React Native courses taught by industry professionals. These courses often include hands-on projects to reinforce your learning.
- YouTube Channels and Video Tutorials: Many developers share their knowledge and experience on YouTube. Channels like The Net Ninja, Traversy Media, and React Native School offer video tutorials specifically focused on React Native development.
- Community Forums and Discussion Boards: Join online communities like the React Native subreddit or the Reactiflux Discord server. These platforms provide opportunities to ask questions, seek advice, and engage with fellow developers.
FAQ 4: Can I use React Native for existing native apps?
Answer: Yes! React Native offers the capability to integrate seamlessly with existing native codebases. This means you can gradually introduce React Native components and features into your existing iOS or Android app, without having to rewrite the entire application. React Native's modular approach allows you to choose which parts of your app to build using React Native while maintaining the rest in native code.
FAQ 5: Is React Native suitable for all types of mobile applications?
Answer: React Native is well-suited for a wide range of mobile applications, including but not limited to:
- Consumer-Facing Apps: React Native excels in building visually appealing, feature-rich applications with smooth user experiences. Whether you're creating social media apps, e-commerce platforms, or entertainment applications, React Native provides the tools and performance needed to deliver high-quality experiences.
- Enterprise Applications: React Native is increasingly adopted in the enterprise space. It allows companies to streamline their development process by building cross-platform applications that cater to both iOS and Android users. React Native's ability to integrate with existing native codebases also makes it a compelling choice for enterprise app development.
- Prototyping and MVPs: React Native's rapid development capabilities make it an excellent choice for prototyping and building Minimum Viable Products (MVPs). It allows you to iterate quickly, gather user feedback, and validate your ideas before investing heavily in native development.
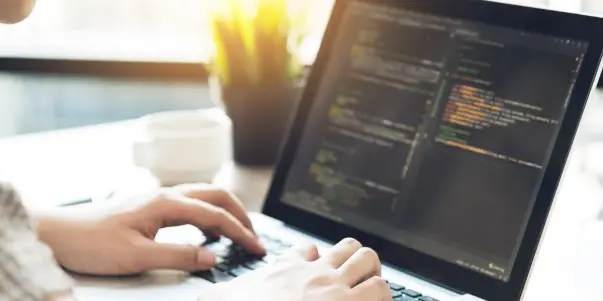
React Native is well-suited for a wide range of mobile applications
Conclusion
In conclusion, becoming a React Native mobile developer opens up a world of opportunities in the ever-growing field of mobile app development. By leveraging the power of JavaScript and React, you can create cross-platform applications with native-like performance and efficiency.
Throughout this guide, we have explored the fundamentals of React Native, the benefits of choosing it for mobile app development, and the essential skills and resources you need to succeed as a React Native developer. We've also answered some frequently asked questions to address common concerns and provide clarity on various aspects of React Native development.
Remember, the key to mastering React Native lies in a combination of theoretical knowledge and practical experience. Continuously update your skills, stay informed about new developments in the React Native ecosystem, and never stop exploring and building projects to enhance your expertise.
So, are you ready to embark on your journey as a React Native mobile developer? Embrace the challenges, dive into the vibrant community, and unlock your potential to create innovative and engaging mobile applications that make a difference in the digital world.