Introducing Props in React Native A Comprehensive Guide
React Native has become one of the most popular frameworks for building dynamic and powerful mobile applications. With its ability to create native-like user interfaces and its efficient code reusability, it has gained a strong following among developers. One of the key features that make React Native so versatile and flexible is the use of props. In this comprehensive guide, we will delve into the world of props in React Native, exploring their purpose, functionality, and advanced usage techniques. By the end of this article, you will have a solid understanding of how to leverage props to build dynamic and reusable components in your React Native applications.
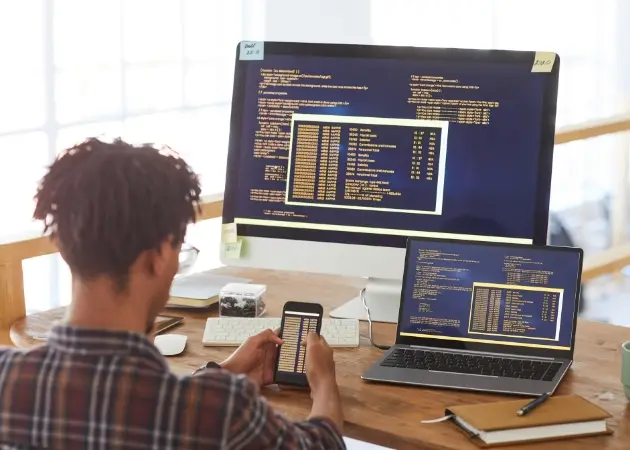
One of the key features that make React Native so versatile and flexible is the use of props
Understanding the Concept of Props: The Building Blocks of React Native Components
Before we dive into the specifics of props in React Native, it's important to understand the concept behind them. Props, short for properties, are essentially parameters that are passed from a parent component to a child component. They allow data to be shared between components, making it easier to build complex and interactive user interfaces.
In React Native, components are the building blocks of an application's user interface. Each component can have its own set of props, which can be accessed and used within the component's code. These props can be anything from simple strings or numbers to more complex data structures such as arrays or objects.
The Role of Props in React Native Components
Props play a crucial role in React Native components by allowing them to communicate with each other. This communication is essential for creating dynamic and interactive user interfaces. For example, a parent component can pass down data to a child component through props, and the child component can then use that data to render specific elements or perform certain actions.
Another important aspect of props is their immutability. Once passed down to a child component, props cannot be changed by the child component. This ensures data integrity and consistency throughout the application.
Creating and Passing Props in React Native
When creating a component in React Native, you can define its props by specifying them as attributes on the component tag. For example:
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
return ;
};
export default ParentComponent;
In this example, name
and age
are props being passed to the ChildComponent
. These props can then be accessed within the ChildComponent
.
Props can also be functions, allowing parent components to pass down functionality to child components. This is commonly used for handling events or updating state in the parent component. For instance:
// ParentComponent.js
import React, from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return ;
};
export default ParentComponent;
In this example, the incrementCount
function and the count
state are passed as props to the ChildComponent
, allowing it to update the count in the parent component when needed.
Passing Data Between Components: Harnessing the Power of Props
As mentioned earlier, one of the key functions of props is to enable data sharing between components. This allows for more dynamic and interactive user interfaces, as well as easier management of data within an application.
One-Way Data Flow in React Native
React Native follows a one-way data flow architecture, which means that data flows from parent components to child components through props. This ensures that data remains consistent and predictable throughout the application.
For example, if we have a parent component that holds a state variable called count
, we can pass this variable down to a child component through props. The child component can then use this prop to display the current count or perform any actions based on the count value.
Passing Functions as Props
In addition to passing data, props can also be used to pass functions between components. This is especially useful when we want to trigger an action in a parent component from a child component.
For example, if we have a button component that needs to update the count variable in the parent component, we can pass down a function that updates the count as a prop. The child component can then call this function when the button is pressed, effectively updating the count in the parent component.
Prop Drilling: A Potential Pitfall
While props are a powerful tool for passing data and functions between components, they can also lead to a phenomenon known as prop drilling. This occurs when props need to be passed through multiple layers of components before reaching the desired component.
Prop drilling can make code more complex and difficult to maintain, as well as decrease performance. To avoid this, we can use state management libraries such as Redux or MobX to handle data at a higher level in the application.
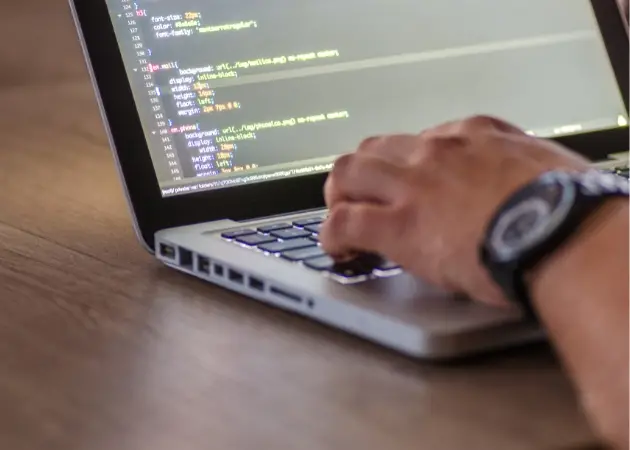
Prop drilling can make code more complex and difficult to maintain
Creating Customized Components with Props: Enhancing Reusability and Flexibility
One of the main advantages of using props in React Native is the ability to create highly customizable and reusable components. By passing different props to a component, we can change its appearance and behavior without having to write separate code for each variation.
Using Props to Style Components
In React Native, styles are typically defined as objects and passed to the style
prop of a component. This allows us to customize the appearance of a component by passing different style props.
For example, if we have a button component, we can pass down a color
prop to change the color of the button based on the context it is being used in. This eliminates the need to create separate button components for different colors, making our code more efficient and reusable.
Conditional Rendering with Props
Props can also be used to conditionally render elements within a component. This is especially useful when we want to show or hide certain elements based on a specific prop value.
For instance, if we have a modal component that can display different types of content, we can pass down a type
prop and use conditional rendering to display the appropriate content based on the type.
Creating Higher-Order Components with Props
Higher-order components (HOCs) are components that take in another component as an argument and return a new enhanced component. Props can be used in HOCs to add functionality or modify the behavior of the wrapped component.
For example, we can create a HOC that takes in a component and adds a loading indicator while data is being fetched. This allows us to reuse this functionality in multiple components without having to write the same code over and over again.
Prop Validation: Ensuring Data Integrity and Consistency in React Native Applications
As mentioned earlier, props are immutable once passed down to a child component. This ensures data integrity and consistency throughout the application. However, it's still possible for unexpected data to be passed down as props, which can lead to errors or unexpected behavior.
To prevent this, React Native provides a feature called prop validation. This allows us to specify the types and shapes of the props that a component expects to receive. If a prop with an incorrect type or shape is passed down, a warning will be displayed in the console.
Using PropTypes for Prop Validation
PropTypes is a built-in library in React Native that allows us to define the types and shapes of our props. It provides a set of validators that we can use to ensure that the props passed down to a component are of the correct type and shape.
For example, if we have a name
prop that should be a string, we can use the PropTypes.string
validator to ensure that only strings are passed down. Similarly, if we have a user
prop that should be an object with specific properties, we can use the PropTypes.shape()
validator to define the shape of the object.
Custom Prop Validation with PropTypes
In addition to the built-in validators, PropTypes also allows us to create custom validators for more complex prop types. This is useful when we have props that require specific validation logic that cannot be achieved with the built-in validators.
For instance, if we have a password
prop that needs to meet certain criteria such as minimum length and special characters, we can create a custom validator to check for these conditions before allowing the prop to be passed down.
Advanced Prop Usage Techniques: Unlocking the Full Potential of Props
While props are primarily used for passing data and functions between components, there are some advanced techniques that can help us unlock their full potential. These techniques allow us to use props in more creative and powerful ways, making our code more efficient and reusable.
Destructuring Props
In React Native, we can use object destructuring to extract specific props from the props
object. This allows us to access props directly without having to use this.props
every time.
For example, instead of using this.props.title
, we can destructure the title
prop and use it as a variable within the component's code. This not only makes our code more concise but also improves performance by avoiding unnecessary lookups.
Using Default Props
React Native allows us to define default values for props in case they are not passed down from a parent component. This ensures that our components have the necessary props to function properly, even if some props are not explicitly passed down.
To define default props, we use the defaultProps
property in our component class. For example, if we have a name
prop that should default to “John Doe” if not passed down, we can write:
static defaultProps = {
name: "John Doe"
}
Props in ReactJS vs. React Native: Exploring Key Similarities and Differences
React Native is built on top of ReactJS, which means that many of the concepts and features in ReactJS also apply to React Native. However, there are some key differences between how props are used in these two frameworks.
Similarities between Props in ReactJS and React Native
The basic concept of props remains the same in both ReactJS and React Native. They are used to pass data and functions between components, allowing for more dynamic and interactive user interfaces.
Both ReactJS and React Native also use PropTypes for prop validation, although the syntax may differ slightly. The same validators such as PropTypes.string
and PropTypes.shape()
can be used in both frameworks.
Differences between Props in ReactJS and React Native
One of the main differences between props in ReactJS and React Native is the way they are defined. In ReactJS, we use the props
keyword within a functional component to access props, while in React Native, we use this.props
within a class component.
Another key difference is the way styles are applied to components. In ReactJS, styles are typically defined using CSS or inline styles, while in React Native, styles are defined as objects and passed to the style
prop of a component.
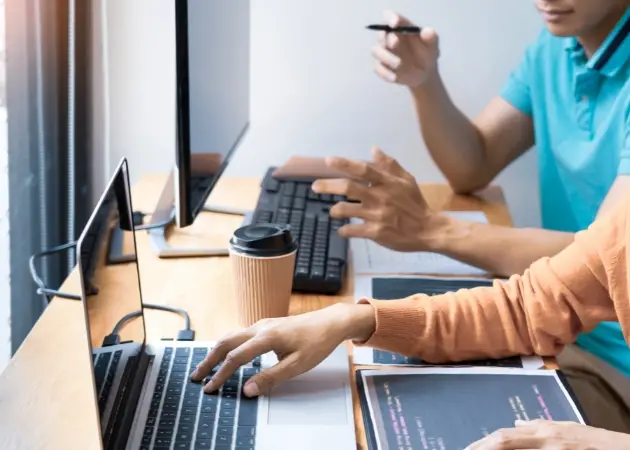
there are some key differences between how props are used in these two frameworks
Leveraging Props for Effective Data Management in React Native Applications
Data management is a crucial aspect of any application, and React Native provides several tools to help us manage data effectively. Props play a significant role in this process by allowing us to pass data between components and trigger actions based on that data.
Using State with Props
State is a fundamental concept in React Native and is often used in conjunction with props to manage data within an application. By passing down state variables as props, we can update the data in a child component based on user interactions or other events.
For example, if we have a form component that needs to update its state when a user enters text in an input field, we can pass down the state variable and a function to update the state as props. This allows us to manage the form's state at a higher level in the application, making it easier to track and update.
Redux and Props
Redux is a popular state management library for React Native that allows us to store and manage application state in a central location. While Redux has its own way of managing data, props can still be used to pass data between components and trigger actions in Redux.
For instance, we can use the connect
function from the react-redux
library to connect a component to the Redux store and pass down data and functions as props. This allows us to access and update the Redux store from within our components, making data management more efficient and organized.
Real-World Examples of Props in Action: Building Practical React Native Components
Now that we have a solid understanding of props in React Native, let's look at some real-world examples of how they can be used to build practical and dynamic components.
A navigation bar is a common feature in mobile applications, and with props, we can create a highly customizable and reusable navigation bar component. By passing different props such as title
, icon
, and onPress
to the component, we can create multiple variations of the navigation bar without having to write separate code for each one.
navigateToScreen("Home")}
/>
A Custom Button Component
Buttons are another essential element in mobile applications, and with props, we can create a custom button component that can be easily customized and reused throughout the application. By passing props such as color
, title
, and onPress
to the component, we can create buttons with different styles and functionality without having to write separate code for each one.
handleFormSubmission()}
/>
A Modal Component with Different Content Types
Modals are a great way to display additional information or actions within an application. With props, we can create a modal component that can display different types of content based on a type
prop. For example, we can have a modal that displays a confirmation message when the type
is set to “confirm” and a form when the type
is set to “form”.
Conclusion: Mastering the Art of Props for Dynamic and Reusable React Native Applications
In this comprehensive guide, we have explored the concept of props in React Native, their purpose, and how they can be used to build dynamic and reusable components. We have also looked at advanced usage techniques and real-world examples of props in action.
By mastering the art of props, you can take your React Native applications to the next level, creating powerful and interactive user interfaces that are easy to maintain and scale. So go ahead and start harnessing the power of props in your React Native projects today!